I needed to change the author link in WordPress post meta. Most of the sites I work on are single author websites, so the Author page is just a copy of the blog page.
I used to just use Yoast SEO to redirect the Author page to home. That's ok, but it seems a bit of a waste, when you could link to the About page, or just remove the link entirely.
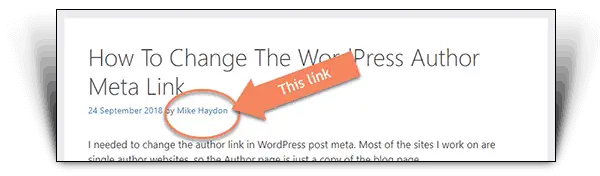
The Post meta is controlled by the single.php file in your theme. Each theme has a different way to manipulate it. But I wanted something simple and quick.
So how do you change the WordPress Author meta link? Add a filter to 'author_link' and return the url you want to use instead of the default.
The simplest code implementation looks like this:
add_filter('author_link', 'my_custom_author_link');
function my_custom_author_link($link)
{
return home_url('/about/');
}
Whatever you put after return is the url that will be output to the post meta.
This code will work on all properly coded themes.
This will apply to every call to get_author_posts_url. In 99% of cases, that will only be on the post information. Be sure to check your implementation if you run say a membership site or do some cool, custom coding on the site.
If you try to return false or otherwise try to return something blank, you won't get rid of the link. It'll just go to the homepage.
How Does This Work?
When the theme calls get_author_posts_url($author_id), it applies the 'author_link' filter, passing in the $link, $author_id and $author_nicename.
You can see from the reference how it constructs the link.
If you wanted to conditionally return different links for different authors, your add_filter and function lines would look like this:
add_filter('author_link', 'my_custom_author_link', 10, 3);
function my_custom_author_link($link, $author_id, $author_nicename) {}
It's important to have the 10 in there, because that denotes the order of the functions running, also known as "priority". You don't need to know anything more about it to get this to work. Just make sure the 3 goes in as the fourth item of the add_filter and matches the same number of arguments passed into the function.
Alternative Implementations
You could also do the add_filter line like this:
add_filter('author_link', 'my_custom_author_link', 10, 1);
You might see this implementation in different guides. It's not necessary if you're only using the first argument, like we are. Your code will work either way.
Some things your code could return are:
- home_url(); // straight to home
- "#"; // best option if you want to remove the link using this method
- "http://www.otherwebsite.com"; // if you wanted to link your author to an external site, like a blog or social media profile.
The last one I wouldn't recommend, unless it was a site you own. If you're doing to social media, you'd be better to setup a page with all your social media links on and a bit about you, then link to that.
Remember that you have to return it, so the get_author_posts_url() has something to return.
Thanks! but how to change the link for a specific author?
Just edit the user's Website field in their profile in the Admin area. Or did you mean how to change it programmatically?
Thanks. It was useful. I was stuck with this and I knew it's simple. But I missed it everytime. After reading this, It was like I just saw it.