Astra is a popular WordPress theme, that I use on many of my sites. It's lightweight and looks great, but sometimes you just want to customize it more than it normally allows. Thankfully it has plenty of hooks.
By default, the post meta in Astra looks like this:
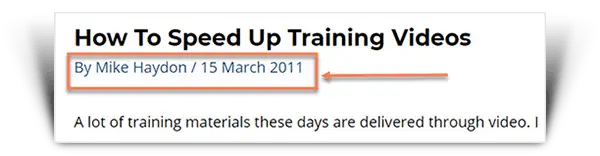
How to customize the post meta in Astra:
- Go to the standard WordPress Customizer (link in your menu bar)
- Navigate to Blog -> Single Post
- Click the eye icon to make elements visible
- Drag the elements to reorder them
To get the post meta to look the way I have in the image above, I've just got Author and Publish Date selected. The slash in between is automatically inserted.
If you're trying to move the post meta, instead of changing it, check out our tutorial on moving Astra's post meta.
How to make Astra post meta elements visible
You can choose to show or hide Astra post meta elements through the Customizer.
In the Customizer, go to Blog -> Single Post to edit the meta for single posts or to Blog -> Blog / Archive to edit the meta on category & tag pages and the home page if it's not set to a static page.
Under Meta, click the eye icon to hide an element.
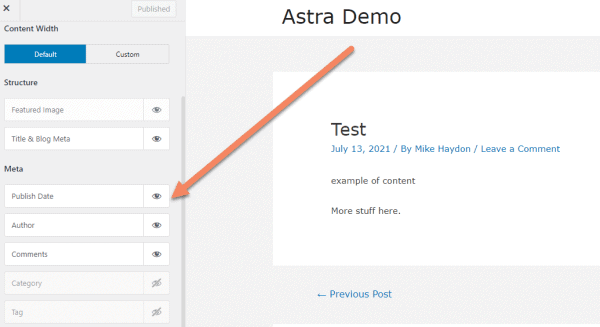
Click a crossed out eye to make an element visible.
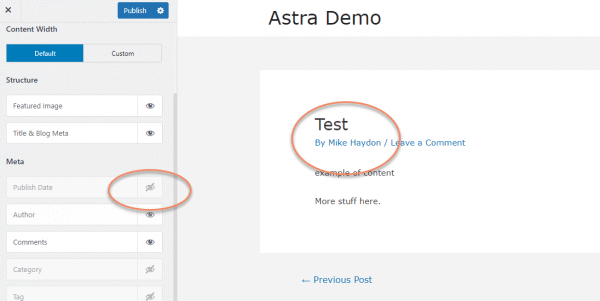
How to reorder Astra post meta items
To reorder the elements in Astra's post meta, go to Blog -> Single Post or Blog -> Blog / Archive in the WordPress Theme Customizer.
Under Meta, you can click and drag any of the elements to reorder them.
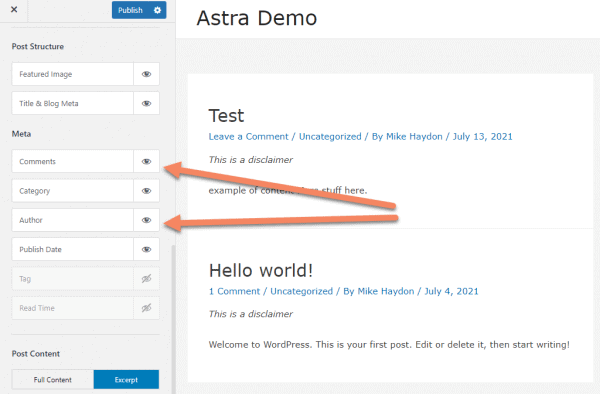
You should see the display refresh as you're working.
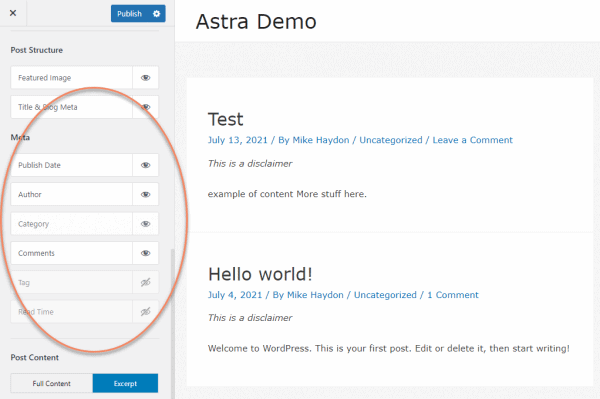
You can also reorder hidden elements, but obviously that will have no effect on the layout unless you make them visible.
How To Change The Slash That Astra Automatically Adds To Post Meta
The slash that Astra automatically adds between the elements of the post meta is usually fine, but sometimes you want something else.
How do you change the slash that Astra automatically adds to the post meta? Add a filter to astra_single_post_meta and astra_blog_post_meta that fetches astra_get_post_meta with your desired separator.
This is the code to make that happen:
add_filter( 'astra_single_post_meta', 'custom_post_meta' );
add_filter( 'astra_blog_post_meta', 'custom_post_meta' );
function custom_post_meta( $old_meta )
{
$post_meta = astra_get_option( 'blog-single-meta' );
if ( !$post_meta ) return $old_meta;
$new_output = astra_get_post_meta( $post_meta, ":" );
if ( !$new_output ) return $old_meta;
return "<div class='entry-meta'>$new_output</div>";
}
You put that code into the functions.php file of your child theme. Never edit the Astra files directly.
You want to hook into both astra_single_post_meta and astra_blog_post_meta so that the changes you make apply to both the single page and other pages like the blogroll and search page. If you just want to change it on the single posts, just use astra_single_post_meta.
The call to astra_get_option() pulls in the Customizer settings you did above.
After error checking, that then gets passed into astra_get_post_meta(), which constructs the post meta as a string. The second argument is the new separator, in this instance a colon, but you can do any character or nothing.
How to add custom html separators
You can even pass html in as the separator, maybe something like a Font Awesome tag. Since Astra doesn't come with Font Awesome installed, you can use something like the Better Font Awesome plugin instead. You don't need to worry about this if you use a page builder like Beaver Builder or Elementor, like I normally do.
Now it looks like this:
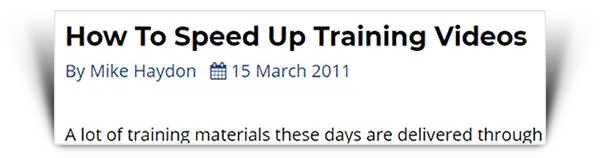
To get it to look like this, I changed $new_output to:
$new_output = astra_get_post_meta($post_meta, " <i class='fa fa-calendar'></i>");
Remember, whatever you put in as the separator will be the separator for each of the elements of the post meta. If you have more elements than what I have here, it could look a little weird.
If you want to have multiple, individual Font Awesome icons in the post meta, you'll have to rewrite astra_get_post_meta(), which you can find in
astra/inc/blog/blog-config.php.
The code above will only change the post meta on single posts. If you want to change the formatting on category pages, use this code as well or instead:
add_filter('astra_blog_post_meta', 'custom_blog_meta');
function custom_blog_meta($old_meta)
{
$post_meta = astra_get_option('blog-meta');
if (!$post_meta) return $old_meta;
$new_output = astra_get_post_meta($post_meta, ":");
if (!$new_output) return $old_meta;
return "<div class='entry-meta'>$new_output</div>";
}
How To Display "Last Modified" Date
Sometimes you want to display the Last Modified or Last Updated date in your post meta. You might want to do this instead of the Published Date or as well as it.
How do you display the "Last Modified" date in Astra? The "Last Modified" date is already in the html of the post meta. All you need to do is unhide it with CSS. Put this code into your custom CSS:
.post .posted-on .updated {
display: inline;
}
To hide the Published Date, put this code into your custom CSS:
.post .posted-on .published {
display: none;
}
How To Modify The Post Meta Date
Lastly, the whole reason I went down this particular rabbit hole was to figure out how to modify the post meta date with my own text. I wanted something that looked like this:
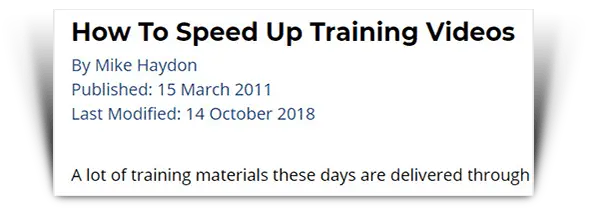
How do you modify the post meta date in Astra? Replicate astra_post_date(), add your own text or formatting and return the output.
To get the post meta looking the way it is in this picture, I used the following code, in combination with the filters and CSS mentioned above:
/* Replaces same named function in /inc/blog/blog-config.php */
function astra_post_date()
{
$format = apply_filters('astra_post_date_format', '');
$published = esc_html(get_the_date($format));
$modified = esc_html(get_the_modified_date($format));
$output = '<p class="posted-on">';
$output .= 'Published: <span class="published" ';
$output .= 'itemprop="datePublished">' . $published;
$output .= '</span><br>';
$output .= 'Last Modified: <span class="updated" ';
$output .= 'itemprop="dateModified">' . $modified . '</span>';
$output .= '</p>';
return apply_filters('astra_post_date', $output);
}
You put that code in your functions.php file of your child theme.
I broke up the $output lines for ease of reading in this tutorial. Change it to suit your code.
Play around with that code to get it looking the way you want. It should be pretty clear what each part does. If you get stuck, just comment below and I'll help you out.
Hi. Did you know how to move post meta to bottom of the post (after content)?
I don't know anything about code.
Hi ASD. I wrote a tutorial on how to do that, which you can find here: https://intelliwolf.com/how-to-move-astra-post-meta/
The custom CSS works, incredible! However, is there a way to show both the last modified date, followed by the original publish date without creating a child theme etc. ? Is there a simpler way to do this using the customizer?
Thanks Sonja. You could do
.posted-on .updated {display: inline;}
But that will just show two dates, without any other text that says one is the modified date and the other is the published date.
To add the extra text you could probably do some jQuery and insert it dynamically, but it's far easier and more stable to create a child theme, in my opinion.
I've done a tutorial on how to create a child theme for any WordPress theme here: https://intelliwolf.com/create-wordpress-child-theme/
It worked! Thank you for the great tutorial. Super easy to do even with my complete lack of skill in this area.
great tutorial!,do you know how we can isolate the tags, we wantthe tags below the post but im having trouble fining the hook for just the tag meta
To isolate the tags, you need to pass that through to the post meta.
So in your theme, wherever you want to display the tags, use this code:
$post_meta = array('tag');
$output = astra_get_post_meta($post_meta);
Hi,
Thanks for this great workaround.
One question though. How do you change the word "By" to "by"?
Hi Mers
Add this code to your child theme's functions.php:
add_filter('astra_default_strings', 'iw_custom_string');
function iw_custom_string($string) {
$string['string-blog-meta-author-by'] = "by ";
return $string;
}
Make sure you leave a space after by, so the words don't run into each other.
Hello, Mike.
Your css helped! Thank you!
How can I center meta line (category, date, author), too?
And also, is there a way to somehow "separate" meta to display "cathegory" above title, and the other meta (date,author) below (as is now), just centered?
I am very new in WP, do not know coding and doing it all by myself.
Thank you.
Hey Iva
The code to center is
text-align: center;
If you've got >div class="entry-meta"> then you would put this into your custom CSS:
.entry-meta {text-align: center;}
Hey Mike!
If only I have read your post yesterday, I would've been able to save 5 hours today! 😅
Btw, is there a way to make the published/last modified date conditional?
Like: show 'last modified date', if there's none (the post hasn't been modified yet), show 'date published'
Is that possible?
Hey Alan. Hopefully it saves you 5 hours tomorrow :)
See in the last part of the tutorial where I've defined $modified? You could do something like this, replacing the lines that start with $output:
$output = '<p class="posted-on">';
if (!empty($modified)) {
$output .= 'Last Modified: <span class="updated" ';
$output .= 'itemprop="dateModified">' . $modified . '</span>';
} else {
$output .= 'Published: <span class="published" ';
$output .= 'itemprop="datePublished">' . $published;
$output .= '</span>';
}
$output .= '</p>';
Hi Mike,
This is brilliant, thank you!!
Is there a way to add the text "updated" before showing the updated date in the meta?
Also, I'm trying to add my gravatar image beneath my post titles in Astra. It appears in the author box beneath my posts, but not next to my author name in the meta. I have been back and forth with Astra's team but haven't found the answer yet.
I don't suppose you can help me with this?
It would be greatly appreciated!
Thanks,
Zoe.
Thanks Zoe :)
For adding "updated", have a look at the last part of the tutorial. You could customize the astra_post_date() function to say whatever you'd like.
The gravatar question is too long to be answered in a comment. I'll do a tutorial on it and update this thread.
Hi Mike :)
Thanks for your help.
That would be great if you could make a tutorial on it. I can't find out how to do it anywhere!
thaaaaaaaaaaaaaaaaaaaaaaaaaaaaank you.
Hi mike,
Thank you for this awesome article! you helped me a lot.
Is it possible to change the slash('/') on the blog posts page?
The code is working only on the single post page.
Yep, I've updated the code in the guide to do that.
Thanks a LOT for this!
hello. thank you for this grate tutorial. is there a way to have number of comments instead of "Leave comment" ?
Astra only displays "Leave a Comment" if there are no comments. If there are comments it displays the number.
If you want to change "Leave a Comment" to something else, like "Go ahead", use this code:
add_filter( 'astra_default_strings', 'iw_blog_comment' );
function iw_blog_comment($defaults) {
$defaults['string-blog-meta-leave-a-comment'] = 'Go ahead';
return $defaults;
}
I've done a tutorial on changing the default text at https://intelliwolf.com/change-astra-theme-default-text/
Hi,
It works very well. I did notice this code only works for the meta in the post itself. When looking at the meta displayed for the post in the "search" section, it remains in the old style. Is there a way to include this so that the meta is displayed in the same fashion?
Kind regards,
Anthony
The hook for what's displayed in the search section is astra_blog_post_meta. So if you wanted the same across both the blog and the search box, you'd add:
add_filter( 'astra_blog_post_meta', 'custom_post_meta' );
That way you're reusing the custom_post_meta function.
Hey Mike!
Great Stuff!
May I know how to change the meta tag font size?
I want a different size in desktop and mobile
Thank You
In your Advanced CSS in the Customizer, add this:
.entry-meta, .entry-meta a {font-size: 20px;}
@media (min-width: 545px) {
.entry-meta, .entry-meta a {font-size: 16px;}
}
Then your desktop meta will be 16px and mobile will be 20px.
Thanks, Mike for this article. It was great to read the article and grab knowledge.
Thank you so much, I've been searching for hours on how to do this (Elementor is so much easier!) The output works brilliantly, but how can I keep everything on one line? Also (if not too much trouble) change color and font weight?
To keep it all on one line, you'd just remove the <br> tags. Those are the line breaks. To change the text color and font weight, you'd connect the CSS to .entry-meta a {}
Hi Mike,
I want to add a horizontal line after the post meta. Can you please tell me how to do that?
You could either create a border-bottom: 1px solid #ddd; or add a <hr> at the end of the custom_post_meta($old_meta) function from the article.
Hey Mike.
Thank you for creating and helping and saving my valuable hours.
I visited your YouTube channel, you're doing good. But please make blogs to YT videos so that people can understand even more clearly.
You're awesome !! Lots of love from India.
This is a life saver! Huge thanks!
I wonder how I can move category names to bottom of posts everywhere and make them label-like look by putting a background color for each category name, also removing the commas between category names.
Is it also possible to leave published date info at the top below the post title and possibly with calendar icon before it?
this is my current site with GeneratePress and I'm trying to move that desing to my new web site with Astra.
https://powerbi.istanbul
Thank you! The CCS in combo worked great to display the date the post was last updated.
Do you also happen to know how I can display meta data before every post like an affiliate disclaimer? I just now switched from Genesis to Astra and in Genesis, I could set meta data for singular content. But I don't know how to achieve that in Astra. Ideally, I'd like my disclaimer to be visible on top of every post, under the title and above the social share icons, basically in between, but NOT to display in the post preview on category pages. In Genesis the meta data disclaimer was visible all the time and it took up a lot of space, also didn't look inviting as the reader got hit with an affiliate disclaimer. If you could point me in the right direction that would be fab. Thank you!
I've added a tutorial on how to do that at https://intelliwolf.com/add-text-to-every-post-astra/