I was building an accordion in Divi, using the standard accordion widget.
Checking it against the Aria Design Patterns for Accordions and the Accordion Example from W3, the Divi accordion module wasn't following the correct labelling requirements.
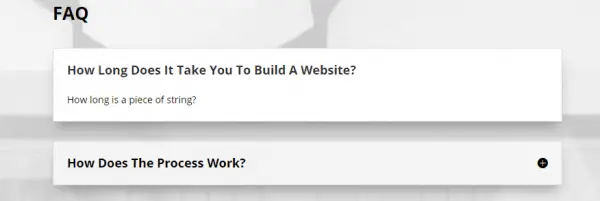
Perhaps by the time you're reading this, it has been fixed. If not, I hope you find this useful.
How to make a Divi accordion accessible: Add a code module to the page containing the accordion that sets the correct roles and aria attributes using jQuery.
If you just want the code, it is:
<script type="text/javascript" async>
jQuery(document).ready(function ($) {
// Add ARIA to accordion
$(".et_pb_accordion .et_pb_toggle_content").attr("role","region");
$(".et_pb_accordion .et_pb_accordion_item").each(function(i) {
var accordionID = "accordion-" + i;
$(this).children(".et_pb_toggle_content").attr({
"id":accordionID,
"aria-labelledby":accordionID + "-heading"
});
var headerText = $(this).children("h3").text();
$(this).children("h3").html(
"<span role='button' id='" + accordionID + "-heading' aria-controls='" + accordionID + "'>" + headerText + "</span>");
});
update_accordion_aria();
$(".et_pb_accordion").on("click",".et_pb_toggle_title, .et_fb_toggle_overlay", function() {
setTimeout(update_accordion_aria, 1500);
});
function update_accordion_aria() {
$(".et_pb_accordion .et_pb_toggle_open h3 span").attr("aria-expanded","true");
$(".et_pb_accordion .et_pb_toggle_close h3 span").attr("aria-expanded","false");
}
});
</script>
This setup assumes you're using H3 for the accordion headings. To make this script work, either use H3 on your accordions or change H3 references in the script to H5 (or whatever heading level you're using).
How to add a code module to Divi
Hover over the accordion module until you see the grey circle with the plus icon. Click that.

Type "code" to find the code module, or scroll through the New Modules until you find it. Click it.
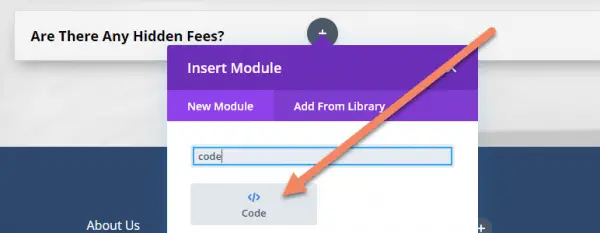
Paste the code from above into the Code area.
Click the green check mark button to save the code.
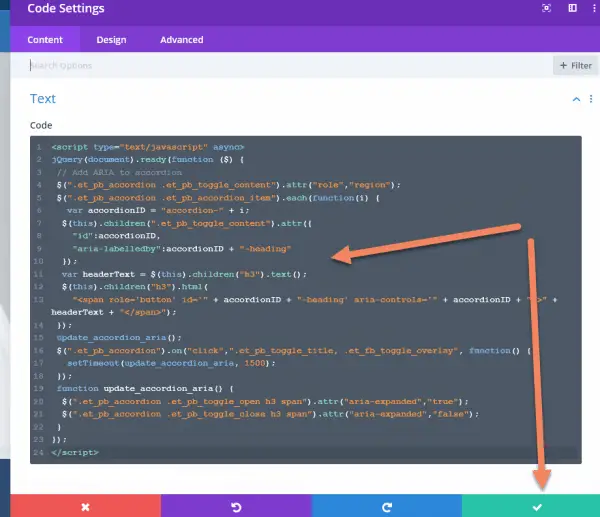
Be sure to save the page.
Before making these change, the outputted code will look something like this:
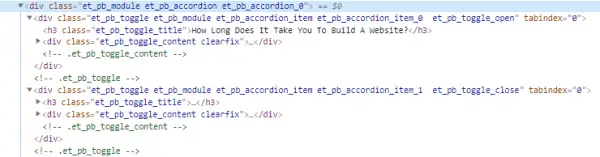
And after implementing the code and refreshing, you should have all the correct elements of an accordion set:
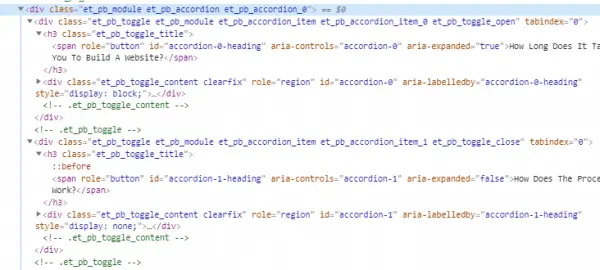
What we're adding
Let's go line by line through the code we added, to see what it does.
$(".et_pb_accordion .et_pb_toggle_content").attr("role","region");
We add role="region" around the text that gets displayed by the accordion.
$(".et_pb_accordion .et_pb_accordion_item").each(function(i) {
var accordionID = "accordion-" + i;
$(this).children(".et_pb_toggle_content").attr({
"id":accordionID,
"aria-labelledby":accordionID + "-heading"
});
var headerText = $(this).children("h3").text();
$(this).children("h3").html(
"<span role='button' id='" + accordionID + "-heading' aria-controls='" + accordionID + "'>" + headerText + "</span>");
});
We cycle through each of the accordion items, connecting the headings and the content.
Each of the content areas get an ID for the heading to reference and they reference the correct heading using aria-labelledby.
We then grab the text from the heading to use in the next step.
Inside the h3 tag, we create a span with the role of "button".
We set the ID of the heading to be different from the ID of the content. This is what the content aria-labelledby will point at.
We then tell the heading which content block to reference, using aria-controls.
update_accordion_aria();
function update_accordion_aria() {
$(".et_pb_accordion .et_pb_toggle_open h3 span").attr("aria-expanded","true");
$(".et_pb_accordion .et_pb_toggle_close h3 span").attr("aria-expanded","false");
}
Next, we set the aria-expanded attribute of the headings, depending on whether the content for that accordion item is open or closed.
$(".et_pb_accordion").on("click",".et_pb_toggle_title, .et_fb_toggle_overlay", function() {
setTimeout(update_accordion_aria, 1500);
});
Finally, we listen for the "click" handler, which is set for the accordion module in Divi/js/custom.unified.js. When that gets triggered, we wait 1.5 seconds and update the aria-expanded values for each of the headings.
We have to wait at least 1 second, because it's based on whether the heading parent has the class et_pb_toggle_open or et_pb_toggle_close and it takes around a second for that to change. I just added half a second to be safe, but if you're not seeing the aria-expanded change, you might want to increase this value.
If you have multiple accordions on the page, this should still be fine, but make sure to test it yourself. You won't need to add the script multiple times.
If you have an accordion on every page, or most pages, it's fine to put this script into the footer scripts from Divi and set it sitewide.
How to change the heading level
This script relies on you using heading level 3, ie H3.
But what if you want to use a different heading level?
Start by changing the heading level in the accordion. To do so, open the individual accordion settings, go to the Design tab, expand the Title Text and select a different heading level.
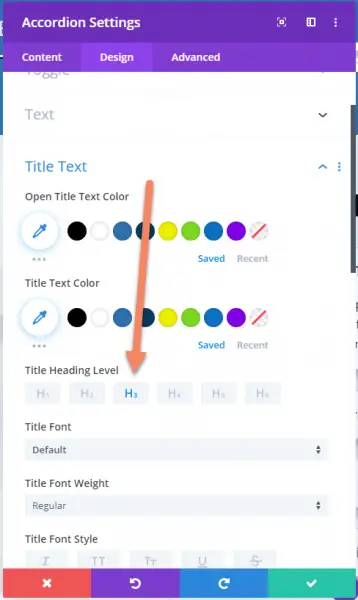
Then in the code you copied from above, change the four references to h3 to the new level. For example, if you changed them to H4, you'd change the references in the code to "h4".
I've highlighted the locations below.
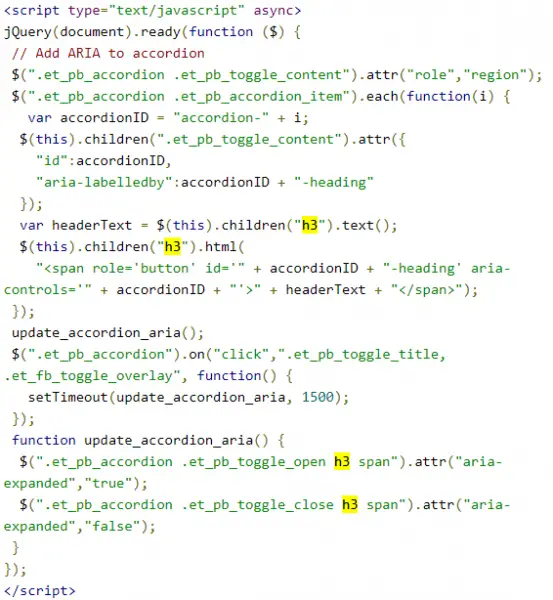
If you don't change all of the references, the code won't work fully.
Hi Mike,
This script is what we need. We have created accessible technology for folks in the US with vision limitations. We need to add an accessible accordion to divi. Not sure why this script isn't working for us. https://menus4all.com/cafe-eclectic-accord-test. I left you a request to help us solve this. - Be Well, Helen
Hi Helen
I'll email you directly, but for anyone else reading this, I think the issue is you're using h5 for your accordion headings. You either need to change the accordion headings to h3, or change the script references from h3 to h5.
Thank you Mike! - If you ever need WCAG testing help reach out. - Helen
Hi Mike !
I hope you are fine !
That script is very interesting, I search the same thing for the "toggle module". We need to add an accessible toggle to divi. Do you have the code for that ?
Thank you so much !
Victoria
Hi Victoria.
I've written a tutorial on that here: https://intelliwolf.com/divi-toggle-module-web-accessible/
You save my life and my time.
thanks a lot for this useful article.
Hello,
This makes sense, but it isn't unfortunately isn't working for me after following all of the directions. In your screenshot of the code inspector, it looks like your headers are already H3s before implementing code, so it's not apparent to me what it exactly this code is remedying for your site.
The code doesn't change the heading levels. The code adds the correct aria labels and roles to the accordion.